Aggregate Errors with Custom Fingerprints

Vu Ngo -
One of Sentry's most useful features is that we aggregate similar events together to create groups of Issues. We determine which errors should be grouped together by fingerprinting them, and, by default, this fingerprinting is done using all lines in the entire stack trace. Should the stack trace not be available for some reason, then we fall back on the exception, template, or message to determine how an error should be aggregated. (See this short guide for more information about our grouping priorities.)
However, you don't have to rely only on our fingerprinting methods to determine how your errors should be grouped. You can also create your own custom fingerprinting on the client side, which is given priority over our own.
There are a couple of options that allow you to set a custom fingerprint, but the most efficient method is to use the dataCallback function to force fingerprints on errors matching a specific pattern.
To consider this, let’s inspect the standard Raven configuration.
Now let’s add dataCallback to the config. The data object that’s passed to the dataCallback function contains all of the information, including the custom fingerprint, that Sentry will receive when an event is sent.
To add a custom fingerprint to the data object, all you need to do is pass an array to the fingerprint attribute of the data object:
data.fingerprint = [“CUSTOM_FINGERPRINT”]
In this particular use case, we want to check that the error message matches a regular expression (e.g. /^SecurityError: Blocked a frame/
) before setting the custom fingerprint. The error message could also be found in the data object:
data.exception.values[0].value
Custom Fingerprint Example:
Now let’s put it all together.
Once the event has been sent, check the JSON payload to verify that the custom fingerprint was received by Sentry.
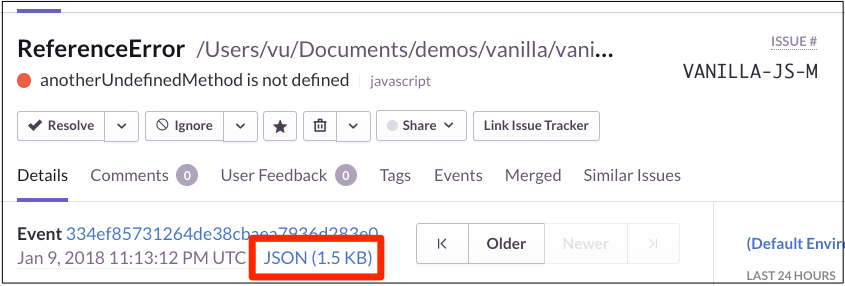
If it has, then you’re all set. Errors that don’t match the custom fingerprint will continue to be grouped according to our standard grouping priorities. Have questions? Our support engineers are here to answer them.