Sentry's Pinia Integration for Vue and Nuxt Error Tracking
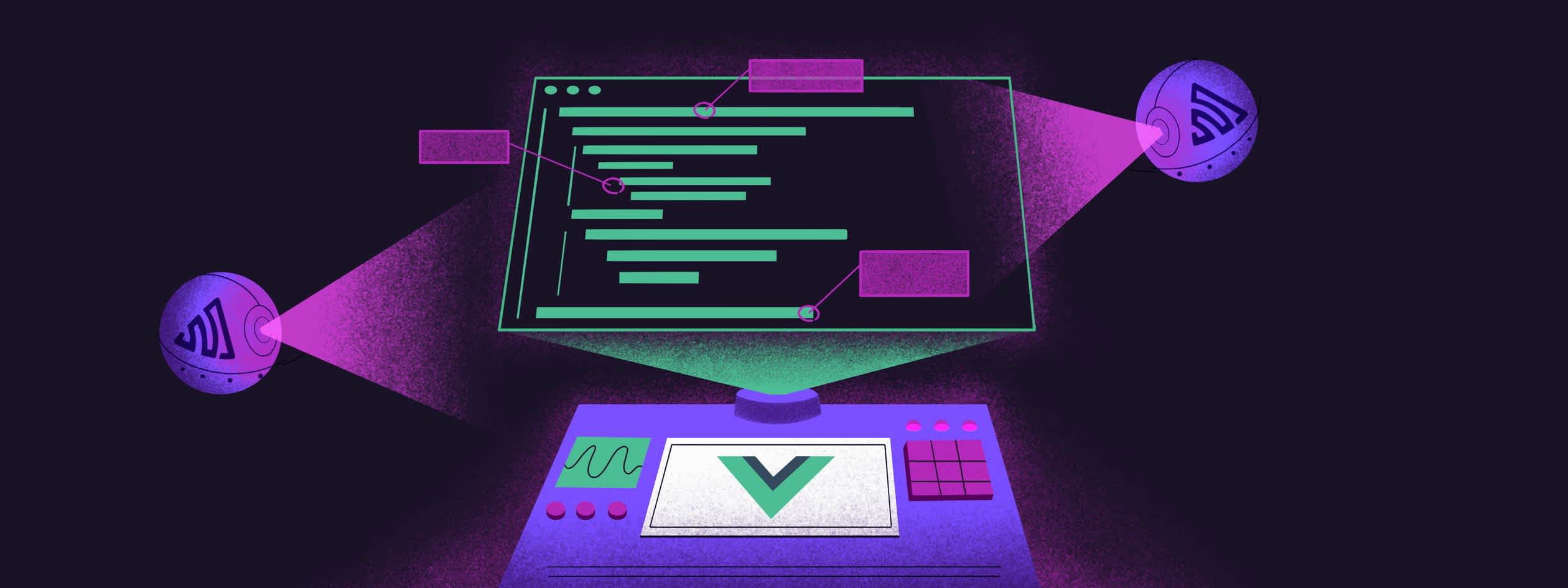
ON THIS PAGE
- Debug shopping cart issues faster with Sentry
- Required modals introduce complex bugs
- Beyond State: Sentry’s Existing Vue & Nuxt Features
- Getting Started with the Pinia Integration
When debugging issues in production, context is everything. While Sentry already provides rich error data like stack traces, breadcrumbs, and user information, understanding the application state at the time of an error can still help reproduce, fix and ship quickly.
Sentry’s Pinia integration solves this by automatically capturing Pinia state wherever errors occur. Now you get the complete picture of your Vue or Nuxt application's state at the moment things went wrong.
Let's look at how to implement this in both Vue and Nuxt applications.
Meet the Pineapple Paradise store, inspired by Pinia's pineapple logo 🍍.
This Vue app demonstrates state management for a shopping cart using Pinia and behaves as you would expect online stores to behave: users browse items and add them to a shopping cart. Pinia manages the state of the shopping cart.
Here is a simplified version of the state management code for the shopping cart:
src/stores/cart.js
Click to Copyimport { defineStore } from 'pinia' export const useCartStore = defineStore('cart', { state: () => ({ items: [] }), actions: { addItem(item) { this.items.push(item) } } })
Initializing the Sentry integration for Pinia is as simple as using Sentry with any of our other frameworks. In your main.js
file, simply import the Sentry Vue SDK, call init
with the options you need, and then call pinia.use(createSentryPiniaPlugin())
. Here is an example:
src/main.js
Click to Copyimport { createApp } from 'vue' import { createPinia } from "pinia"; import { createRouter, createWebHistory } from 'vue-router' import * as Sentry from '@sentry/vue' import { createSentryPiniaPlugin } from "@sentry/vue" import App from './App.vue' //…router config…// const pinia = createPinia(); const app = createApp(App) Sentry.init({ app, dsn: SENTRY_DSN, integrations: [ Sentry.browserTracingIntegration({ router }), ], }) pinia.use(createSentryPiniaPlugin()); app.use(pinia) app.use(router) app.mount('#app')
Now, imagine that when a user attempts to checkout with their favorite pineapple-related items, your site crashes and you lose a customer. Right when your users encounter the issue, the error event is automatically sent to Sentry with all relevant context. Relevant context, in this scenario, includes:
Stack Trace: Within minutes of opening the issue, developers can find the root cause, with Sentry linking the error directly to the relevant source code using sourcemaps.
Pinia State: The state of your application at the moment of the error is also automatically attached to your issue. Offering insights into how users state might have contributed to the issue.
Here is what it looks like when an error occurs and you open the associated issue in Sentry to begin debugging:
Leveraging the rich code-related data, along with application state, enables you to not only understand and resolve issues faster, but confirm issues were resolved by testing with the same or similar state.
Every website has a modal these days, but sometimes the modal is a required and critical step for our users. For our second example, we have a very simplified Nuxt-powered website where users can book appointments, but only if they have accepted some terms and conditions.
In this example, Pinia is used to manage the state for the visitor, whether they have accepted the terms and conditions, and (therefore) if they can book an appointment.
Here is a simplified version of the state management code:
src/stores/terms.js
Click to Copyimport { defineStore } from 'pinia' export const useTermsStore = defineStore('terms', { state: () => ({ accepted: false }), actions: { accept() { this.accepted = true } } })
Initializing the Sentry integration for Pinia for a Nuxt application is similarly easy compared to the Vue example. To capture Pinia state data, add the piniaIntegration
with usePinia()
to the integrations array of your client-side Sentry configuration:
sentry.client.config.ts
Click to CopySentry.init({ dsn: SENTRY_DSN, integrations: [Sentry.piniaIntegration(usePinia())], });
In this example I have also set tags on the events I send to Sentry. Tags allow you to filter your issues feed, filter traces, and analyze performance data in Sentry. Below you can see how you can set the tags based on your Pinia state management.
user.js
Click to Copyimport { defineStore } from 'pinia' import * as Sentry from "@sentry/nuxt" export const useUserStore = defineStore('user', { state: () => ({ hasAgreedToTerms: false, hasSeenModal: false, hasDeniedTerms: false }), actions: { setSentryTags() { // Set all state properties as Sentry tags Object.entries(this.$state).forEach(([key, value]) => { Sentry.setTag(key, String(value)) }) }, acceptTerms() { console.log('Previous state:', { hasAgreedToTerms: this.hasAgreedToTerms }) this.hasAgreedToTerms = true this.hasSeenModal = true this.hasDeniedTerms = false console.log('Updated state:', { hasAgreedToTerms: this.hasAgreedToTerms }) this.setSentryTags() }, denyTerms() { console.log('Previous state:', { hasAgreedToTerms: this.hasAgreedToTerms }) this.hasAgreedToTerms = false this.hasSeenModal = true this.hasDeniedTerms = true console.log('Updated state:', { hasAgreedToTerms: this.hasAgreedToTerms }) this.setSentryTags() } } })
I set tags based on whether the user:
Saw the modal window
Agreed to terms and conditions
Denied the terms and conditions
In this example, we're using tags because this is a critical flow for users of this application and it's not always obvious what information will be helpful during debugging. These tags will at least provide enough information to know where we need to debug further, depending on if the user was accepting or declining the terms and conditions.
In this scenario, when you debug issues with Sentry you will be able to verify the state your users had at the time the error was triggered. When debugging this issue in particular, I focused on leveraging:
Sentry Issue with Stacktrace: Same as before your Sentry issue will link to your source code, with sourcemaps uploaded as part of our Nuxt SDK.
Custom Tags: Custom tags will allow you to filter events, create dashboards, and create alerts
Pinia State Attachment: Finally the user’s state is again attached to the issue in Sentry
Here is what it looks like when an error occurs and you open the associated issue in Sentry to begin debugging:
Before we wrap up, it’s worth touching on some of the existing Sentry features that help with Vue and Nuxt application monitoring:
Performance Monitoring and Insights: From performance issues to specialized insights for frontend, backend, mobile and AI, Sentry captures it all.
Session Replay: Session Replay offers a video-like reproduction of user actions, further complemented by user feedback integration.
User Feedback: It has never been easier to collect user feedback directly in your application.
Vue-specific features: Sentry provides integrations specialized to Vue features besides Pinia as well. Support for component tracking, the Vue router, and mutliple Vue apps, see our Vue and Nuxt docs respectively to learn more.
Ready to get started? Check out our detailed documentation for:
Both guides include configuration options to customize the integration, including features to protect sensitive information from being captured. If you're working with React and Redux instead, we've got you covered with our Redux integration as well.
Try it for yourself, and if you have any feedback please feel free to reach out on Discord or by creating an issue for Sentry's JavaScript SDK in GitHub.