Automating Sentry Releases with CircleCI
Continuous integration tools like CircleCI let developers automate builds and tests, so that teams can merge changes into their codebase quickly and frequently. In this article, we’ll take a look at how to combine Sentry’s command line interface with CircleCI to automatically create Sentry releases. This will unlock some of our best features, like identifying suspect commits that likely introduced new errors, applying source maps to see the original source code within Sentry, and more.
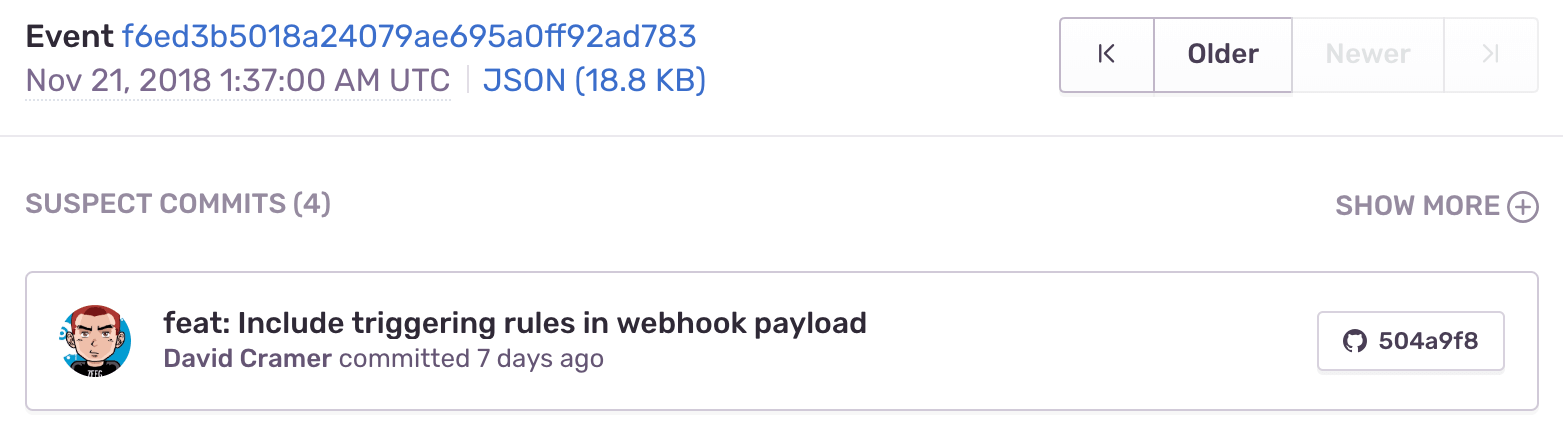
Learn more about Sentry releases here.
Making a CircleCI configuration file
If you’re new to CircleCI, follow their “getting started” guide — you’ll need to create a CircleCI account and link up your project’s repository, which can be hosted on GitHub or Bitbucket.
Your CircleCI build process is defined in a config.yml
file, which needs to be in a .circleci/
directory. Here’s an example of a bare-bones config file:
version: 2
jobs:
build:
docker:
- image: circleci/node:4.8.2 # the primary container, where your job's commands are run
steps:
- checkout # check out the code in the project directory
- run: echo "Hello world!" # run the `echo` command
CircleCI config files contain a list of jobs, which contain a series of steps to run. (Note: If you’re not using their workflows feature, at least one job needs to be named build
.) Each job can also be executed in different environments: within a Docker image or within a virtual machine image for Linux, Mac, or Windows. The example above uses circleci/node:4.8.2
, one of CircleCI’s predefined Docker images.
The steps
value contains a list of key/value pairs, where the key defines the type of step. For example, the “checkout” step is a special pre-defined action that checks out the code from your project’s repository, automatically adding the required auth keys to interact with GitHub and Bitbucket over SSH.
To run Bash commands, you can either use a series of run
steps, or you can include multiple commands within a single step:
steps:
- checkout # check out the code in the project directory
- run: |
echo "Hello..."
echo "...World!"
Note: CircleCI runs each step in a new shell, so environment variables are not shared across steps.
To run a CircleCI job only for certain branches, you can set the branches
key to choose specific branches — for example, to run a job only when a commit is pushed to the master
branch:
build:
branches:
only:
- master
Creating a Sentry Internal Integration
Our Integration Platform allows developers to connect Sentry with third-party tools — either as Public Integrations that anyone can use, or as Internal Integrations built only for one organization to combine Sentry with their internal tools and custom workflows.
To create a new Internal Integration, navigate to Settings > Developer Settings > New Internal Integration within Sentry. There, you’ll give your new integration a title (for example, “Create Sentry Releases with CircleCI”), choose which permissions to use, and get your token for authenticating with Sentry’s API.
Setting permissions
You can apply different sets of permissions for each integration. For this one, we’ll need Admin
access for “Release” and Read
access for “Organization”:
See our docs page on permissions to learn more about scopes for Sentry’s API endpoints.
Next, click “Save” at the bottom of the page and then grab your token:
In the next section, we’ll use this Internal Integration token to enable CircleCI to authenticate with Sentry’s API when creating a new Release.
Creating a Sentry release with CircleCI
You’ll first need to ensure that Sentry releases are set up for your Sentry project. The simplest way to connect your commit metadata to Sentry is to use our GitHub integration or our Bitbucket integration. (Note that CircleCI currently only supports GitHub and Bitbucket.)
Next, we’ll set a few environment variables to configure the Sentry CLI:
SENTRY_AUTH_TOKEN
- Your Internal Integration token.SENTRY_ORG
- Your Sentry organization slug.SENTRY_PROJECT
- Your Sentry project slug.
To save any sensitive data like the SENTRY_AUTH_TOKEN
, CircleCI provides a page for Build Settings in their admin backend. Any environment variables saved there will be redacted in your logs, appearing as ****
so that private data can’t be revealed accidentally.
To save non-sensitive data in the build environment, use the environment
key to define your environment variables in your config.yml
file:
build:
environment:
SENTRY_ORG: my-org
SENTRY_PROJECT: my-project
Finally, to install the Sentry CLI on CircleCI’s virtual machine and create a new Sentry release, we’ll use the following Bash commands:
run: |
curl -sL https://sentry.io/get-cli/ | bash
export SENTRY_RELEASE=$(sentry-cli releases propose-version)
sentry-cli releases new -p $SENTRY_PROJECT $SENTRY_RELEASE
sentry-cli releases set-commits --auto $SENTRY_RELEASE
sentry-cli releases finalize $SENTRY_RELEASE
Here’s our complete example config.yml
file that will create a new Sentry release every time a commit is pushed to the master branch:
version: 2
jobs:
build:
docker:
- image: circleci/node:4.8.2
environment:
SENTRY_ORG: my-org
SENTRY_PROJECT: my-project
branches:
only:
- master
steps:
- checkout # check out the code in the project directory
- run: |
curl -sL https://sentry.io/get-cli/ | bash
export SENTRY_RELEASE=$(sentry-cli releases propose-version)
sentry-cli releases new -p $SENTRY_PROJECT $SENTRY_RELEASE
sentry-cli releases set-commits --auto $SENTRY_RELEASE
sentry-cli releases finalize $SENTRY_RELEASE
Be sure to check out our documentation for more details about managing Sentry releases.
Testing CircleCI configuration files locally
CircleCI provides a convenient command line interface for testing your config.yml
file locally. YAML syntax errors are much easier to catch by testing your config.yml
file locally, rather than pushing code to your repository and waiting for CircleCI’s servers to run your build each time.
To validate your config file locally, use the validate
command:
circleci config validate -c .circleci/config.yml
And to run a job locally, use the local execute
command:
circleci local execute --job "jobname"
Note: When running a build locally, CircleCI does not import any encrypted variables configured via their UI. They recommend setting those environment variables via the CLI in this format: circleci local execute --job "jobname" --env MY_VAR=MY_VALUE
You can also use git hooks to validate your CircleCI configuration file every time you make a commit; see CircleCI’s blog post for a walkthrough.
Be sure to reference CircleCI’s full configuration syntax guide to troubleshoot any tricky YAML issues.
Verifying that your Sentry releases are working
Push a new commit to the branch specified in your config.yml
file to trigger CircleCI to run your build. You can view details about the build in CircleCI’s UI and see any errors listed there if it fails.
If the CircleCI build succeeded, head over to your Sentry organization and click “Releases” on the left-side menu. You should see a brand new release listed for your project, listing the SHA of your latest commit:
To confirm that new events from your project will be correctly associated with your releases, you can use the Sentry CLI to send an event for your project. If you run this from within your project’s git repository, the current release will be linked to the event automatically:
export SENTRY_DSN=https://mydsnhere@sentry.io/1234
sentry-cli send-event -m "Test event, should be linked to the latest release"
See our docs for more about sending events with Sentry’s command line interface.
After sending the event, check your project’s “Releases” page again in Sentry. On the page for your latest release, you’ll see a new unresolved issue associated with the release:
Go forth and continuously integrate
By combining Sentry’s VCS integrations, Sentry’s command line interface (or REST API), and your preferred CI/CD tools, you can build the application monitoring workflow of your dreams! As always, you are most welcome to post feedback in our forum or ask our support engineers for help.
And if workflow customization is your jam, be sure to check out our Integration Platform for more ways to combine other tools with Sentry.