Breadcrumbs in Python
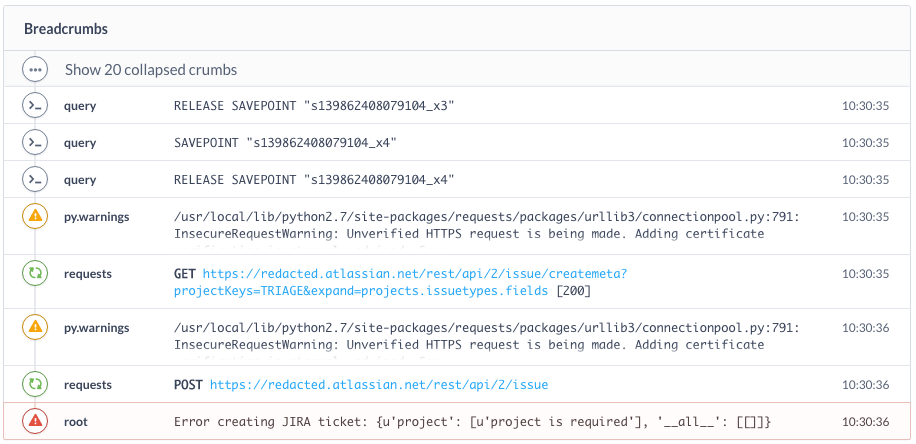
A couple weeks back we announced Breadcrumbs, with full support in our JavaScript error tracking SDK, and the in-progress implementations for other platforms. Today we're going to focus on Breadcrumbs in our Python error tracking SDK, what they capture, and how you get started using them.
What are Breadcrumbs?
Breadcrumbs are a trail of events that occurred in your application leading
up to the primary error. They can be as simple as generic logging messages, or
they can contain rich metadata about the state of your application: network
requests, database queries, UI events, navigation changes, or even earlier
occurring errors.
Breadcrumbs can be incredibly helpful in reproducing the steps that led to the
error you’re debugging. We’ve been dogfooding them here at Sentry for some
time, and they add critical context to errors that a stack trace alone can’t
provide. Once you use them, you won’t go back.
Getting Started
You'll want to ensure you're running the latest version of our Python SDK (raven-python), but anything newer than 5.18 will have some level of support.
There's a variety of events that you might want to capture as part of breadcrumbs, but a common example might be internal RPC calls:
from raven import breadcrumbs
breadcrumbs.record(
data={
'endpoint': rpc.endpoint,
}
category='rpc',
)
You can also pass simple string-based annotations, rather than a JSON blob:
from raven import breadcrumbs
breadcrumbs.record(
message='request to {}'.format(rpc.endpoint),
category='rpc',
)
Automated Instrumentation
Currently Sentry will automatically capture a few things for you:
HTTP requests made using
httplib
,urlib
, orrequests
Logging entries from stdlib's
logging
packageSQL queries from the Django ORM
One common pattern we've already seen is that some loggers might be noisy. Because we instrument and capture all log entries, it often makes sense to filter things out. For example, we might want to disable oauthlib
because it outputs a lot of noise, and potentially sensitive data:
from raven import breadcrumbs
breadcrumbs.ignore_logger('oauthlib')
We'll be evolving this as time goes on, so be on the lookout for more automated instrumentation in the future.
Looking Forward
We're always super excited to bring new levels of data to Sentry. Python error monitoring has been one of our best supported platforms, and we think Breadcrumbs for Python bring it to an entirely new level. That said we're still early in the design, and are rapidly iterating on the concept.