New and Improved Java SDK Released
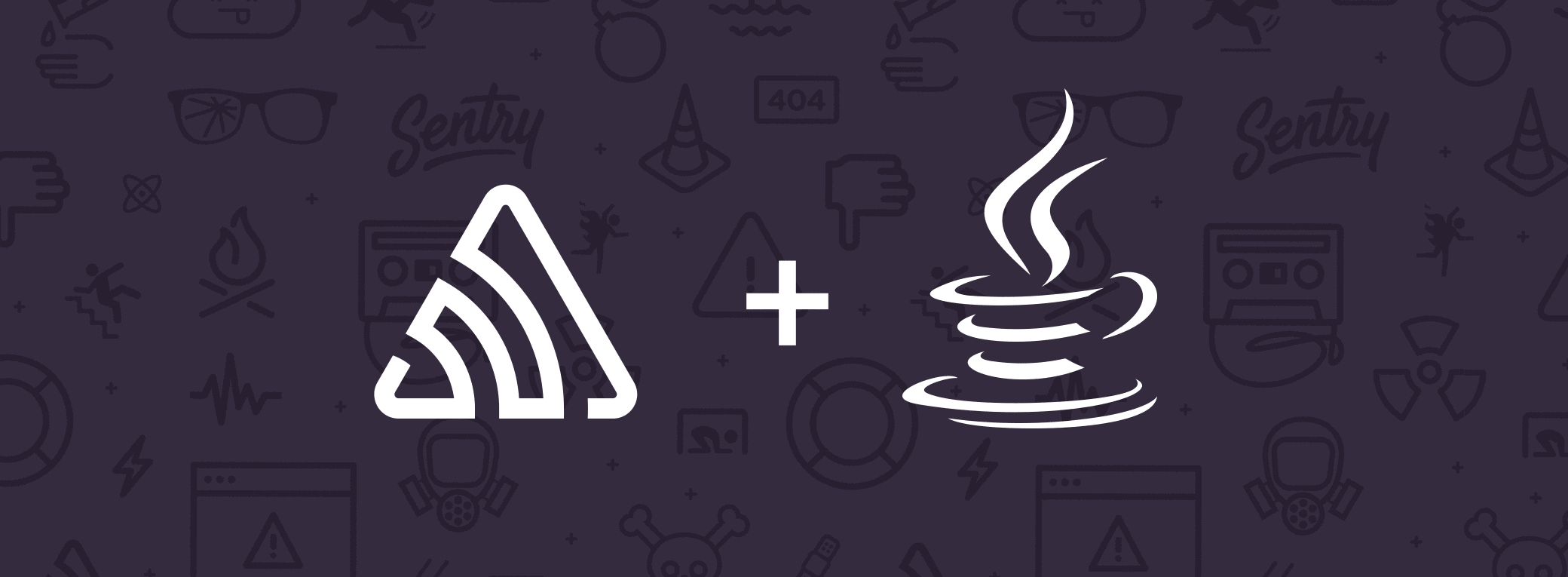
Today we’re announcing the first release of the new and improved Java SDK, sentry-java
. This is a major refactoring (and renaming) of the previous Java client, raven-java
, which aims to add first class support for features like breadcrumbs and integrations other than logging frameworks, such as Android applications.
The original Java SDK, raven-java
, started as a log4j appender that sent error level logs and exceptions to Sentry. From there it was expanded to work with other logging frameworks, but using many of the features outside of a logging framework was either a pain or completely impossible. In addition, the appenders are lazily initialized and so features that need a working Sentry client before an event was sent, such as breadcrumbs, were impossible to use even when combined with a logging framework.
The new Java SDK takes a wholly different approach that focuses on a simple and re-usable Java client that just happens to also be used to implement the logging integrations. For example, add sentry-java
to your dependency manager of choice (note that the group name and artifact have changed):
<dependency>
<groupId>io.sentry</groupId>
<artifactId>sentry</artifactId>
<version>1.0.0</version>
</dependency>
Then use it directly from Java:
import io.sentry.Sentry;
public class Application {
public static void main(String[] args) {
// init manually with a DSN, this can be provided in a number of
// other ways, check the docs!
Sentry.init("your dsn");
// record a breadcrumb
Sentry.record(new BreadcrumbBuilder().setMessage("User made an action!").build());
// set the current user
Sentry.setUser(new UserBuilder().setEmail("hello@sentry.io").build());
try {
runSomething();
} catch (Exception e) {
// send an event to Sentry
Sentry.capture(e);
}
}
}
This example is using a new static API where you don’t have to manage your own client instance. What’s nice is that the logging integrations use the exact same system, so you can set breadcrumbs or the current user and those will be correctly propagated when you log an error.
Note that there are many ways to configure your DSN and other options. Passing the DSN in directly is the least flexible choice and should be a last resort in most cases. See the new documentation for more information on the different configuration methods that are available. Note that configuration of logging integrations has changed – check the docs for your logging framework of choice!
One major benefit of having a plain Java client is that sentry-java
works great on Android. If you use the provided AndroidSentryClientFactory
and pass in your application’s context, the SDK will automatically attach all kinds of useful information to your events such as device name, battery level, OS version, screen resolution, network connectivity, and more.
For example, in your app/build.gradle
:
compile 'io.sentry:sentry-android:1.0.0'
And then in your main activity class:
import io.sentry.Sentry;
import io.sentry.android.AndroidSentryClientFactory;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Context ctx = this.getApplicationContext();
Sentry.init("your dsn", new AndroidSentryClientFactory(ctx));
try {
runSomething();
} catch (Exception e) {
// send an event to Sentry
Sentry.capture(e);
}
}
}
After the initialization above, Sentry will automatically catch unhandled exceptions, and will even cache them to disk for sending later if the user’s network is disabled. See the Android documentation for more information.
The new SDK is also used by our React Native integration on Android for crashes that occur in Java code.
Take a look at the sentry-java project on GitHub to learn more about how things are implemented, and see the documentation for details on using it with Sentry.If you’re not yet a Sentry user, you can try Sentry for free. If that’s not your cup of tea, it’s all open source on GitHub.