Finding and Fixing Django N+1 Problems
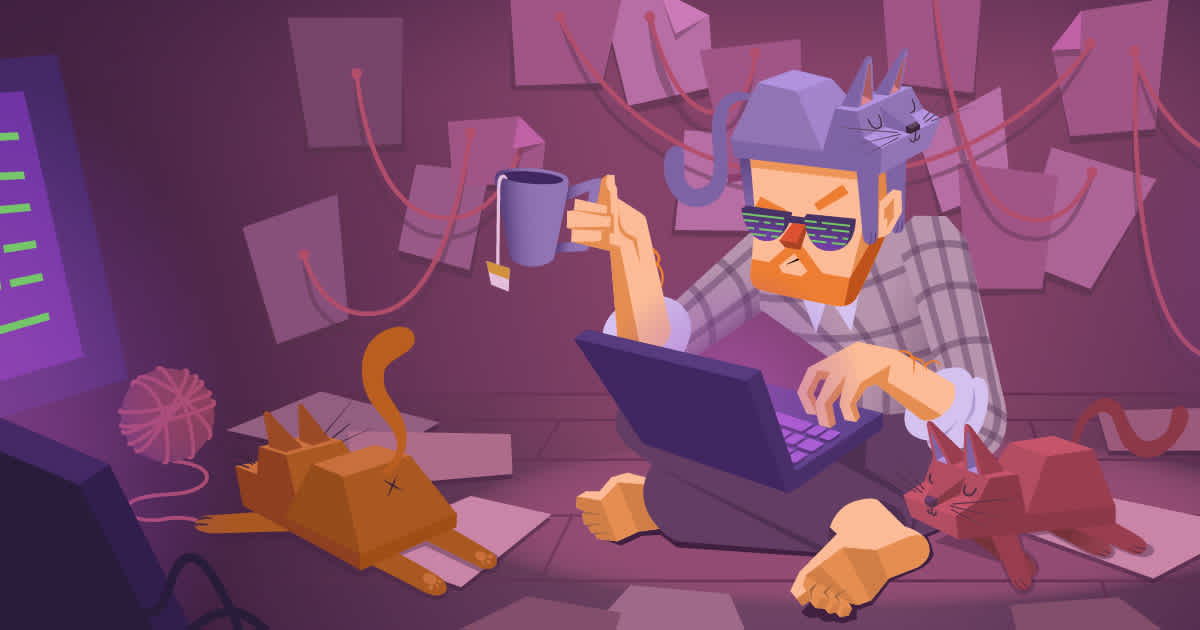
The Django Python framework allows people to build websites extremely fast. One of its best features is the Object-relational mapper (ORM), which allows you to make queries to the database without having to write any SQL. Django will allow you to write your queries in Python and then it will try to turn those statements into efficient SQL. Most of the time the ORM creates the SQL flawlessly, but sometimes the results are less than ideal.
One common database problem is that ORMs can cause N+1 queries. These queries include a single, initial query (the +1), and each row in the results from that query spawns another query (the N). These often happen when you have a parent-child relationship. You select all of the parent objects you want and then when looping through them, another query is generated for each child. This problem can be hard to detect at first, as your website could be performing fine. But as the number of parent objects grows, the number of queries increases as well — to the point of overwhelming your database and taking down your application.
Recently, I was building a simple website that kept track of expenses and expense reports. I wanted to use Sentry’s new Performance tool to assess how the application was performing in production. I quickly set it up using the instructions for Django and immediately saw results.
The first thing I noticed was that the median root transaction was taking 3.41 seconds. All this page did was display a list of reports and the sum of all of the expenses on a report. Django is fast and it definitely shouldn’t take 3.41 seconds.
{{ expense.name }}
Looking at the code I couldn’t see any immediate problems, so I decided to dig into the Event Detail page for a recent transaction.
The second thing I noticed was just how many queries the ORM had generated. It was at this point that I saw that I had a single query to fetch all of the reports and then another query for each report to fetch all of the expenses —an N+1 problem.
Django evaluates queries lazily by default. This means that Django won’t run the query until the Python code is evaluated. In this case, when the page initially loads, Reports.objects.all()
is called. When I call {{ report.get_expense_total }}
Django runs the second query to fetch all of the expenses. There are two ways to fix this, depending on how your models are set up: [select_related()]
and [prefetch_related()]
.
select_related()
works by following one-to-many relationships and adding them to the SQL query as a JOIN. prefetch_related()
works similarly, but instead of doing a SQL join it does a separate query for each object and then joins them in Python. This allows you to prefetch many-to-many and many-to-one relationships.
We can update ReportsList to use prefetch_related()
. This cuts the number of database queries in half, since we’re now making one query to fetch all of the Reports, one query to fetch all of the expenses, and then n queries in report.get_expense_total
.
class ReportsList(ListView):
model = Reports
context_object_name = ‘reports’
queryset = Reports.objects.prefetch_related(‘expenses’)
To fix the n
queries from report.get_expense_total
we can use Django’s annotate
to pull in that information before passing it to the template.
{{ expense.name }}
Now the median transaction time is down to 290ms!
In the event the number of queries increases to the point where it could take your application down, try using prefetch_related or select_related. This way, Django will fetch all of the additional information without the extra queries. After doing this, I ended up saving 950 queries on my main page and decreasing the page load by 91%.
To learn more about using Sentry to find and fix Django N+1 issues, get in touch with us or sign up today!