An iOS developer's first impressions of Flutter: Part 2
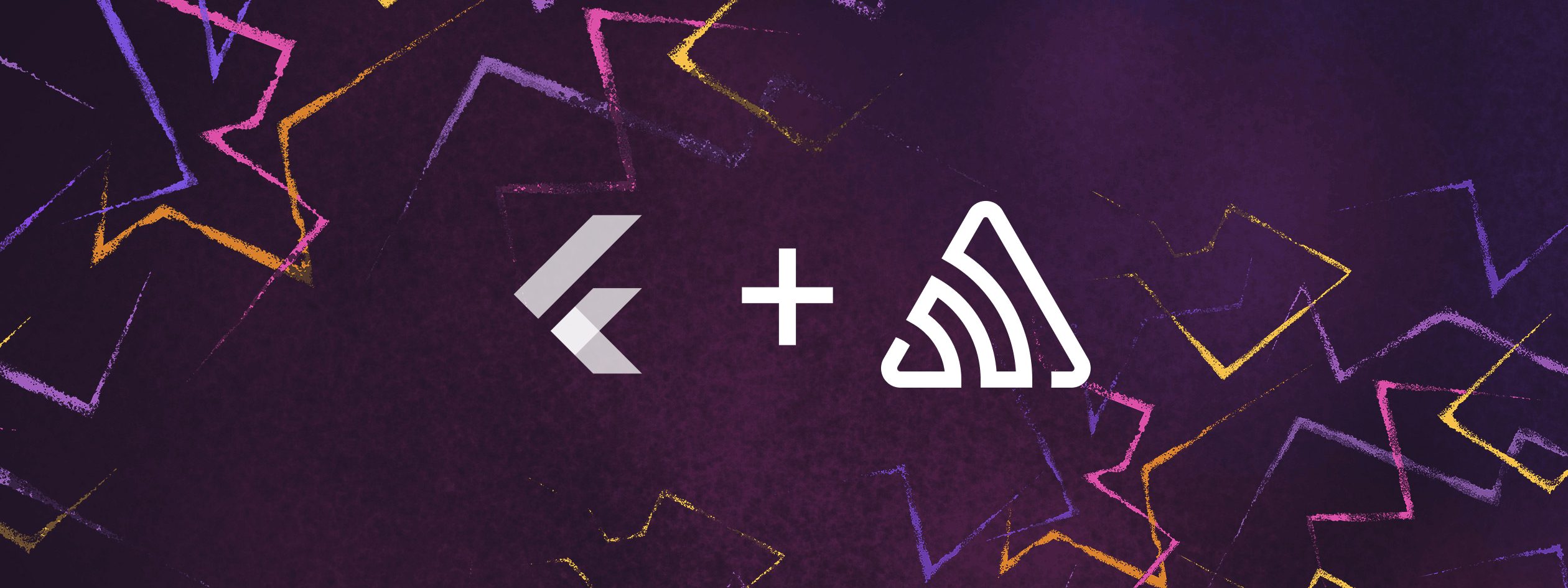
Integrating third party dependencies in Flutter
In the previous article in this series, we looked at my first impressions, as an iOS developer, of Flutter, Google's increasingly popular multiple-platform development tool. Flutter has me quite impressed, but I am curious to know - How easy is it to bring in third party dependencies for multiple platforms?
In my experience working in mobile development, third party dependencies are brought in via platform specific dependency managers - CocoaPods for example in iOS, or the Gradle build system in Android. How can Flutter manage third party dependencies, and make them available for multiple platforms?
Well, it turns out that Dart has its own dependency manager, called the Pub package manager.
When you create a Flutter project, you will find a file called pubspec.yaml is automatically generated in the root folder. This file contains a few basic preference details, such as the package name, description, version number for your application and environment where you can specify the version numbers of the Dart and Flutter SDKs we are expecting our code to use to compile. There is also a section called flutter, where you can specify image assets to include in your app, or custom fonts.
What we are most interested in right now, though, is a section called dependencies. It is here that we can specify third party dependencies needed for the project. In fact - even in a fresh Flutter project you will find you already have dependencies listed here - flutter for the Flutter SDK itself, and cupertino_icons, to give you access to the Cupertino Icons Font for iOS.
You can specify the source for a dependency such as a local path or a git repository, but probably the easiest way to download your dependency is to get it from pub.dev, the official repository for Dart and Flutter, sort of the cocoapods.org of the Flutter world. Though pub.dev might not have direct equivalents for each framework available in cocoapods.org, it does contain many frameworks and libraries with solutions for most common problems.
As a test case for integrating third party dependencies, let's take a look at integrating Sentry in a Flutter project.
Integrating Sentry in Flutter
For those not familiar, Sentry is a mobile performance monitoring tool that tracks any runtime errors in your app. Sentry also helps you to discover performance issues in your app by automatically monitoring how well your app is performing during any potentially slow operations, such as HTTP requests or file operations.
To start off with integrating Sentry into a Flutter app, I search for Sentry in pub.dev.
At pub.dev, the sentry package is actually listed under 'Top Dart packages', but the description does warn you that "For Flutter consider sentry_flutter instead". It looks as though if you are using pure Dart, you should use the sentry package, but if you are working with Flutter, it might be best to check out the sentry_flutter package. There, as with all package listings, you'll find an 'installing' tab which gives you specific advice as to best approach for installing the package.
That said, for most (if not all?) packages, the instructions for adding the package seem to be pretty much the same.
You'll need to open the Terminal. If you are running Android Studio, you'll find the Terminal is accessible right there in the interface - there's a Terminal tab at the bottom left of the interface.
There you'll want to add the package listing to your app, by typing the following:
flutter pub add [package name]
For sentry_flutter for example, you'll want to type:
flutter pub add sentry_flutter
This will automatically add the latest version of the package to your app. If you have your pubspec.yaml file open, you might need to close and reopen it to see the changes.
You will probably now see something like the following line, in the dependencies section:
`sentry_flutter: ^6.6.3`
In addition to adding this dependency line to your pubspec.yaml file, the 'flutter pub add' command also automatically runs the 'flutter pub get' command, which will download any dependencies in your project.
The version number you see of course may change in the future. If you want to update your dependencies to the latest version, you can run the 'flutter pub upgrade' command.
You can check that the sentry package is now accessible in code, by simply importing it in the main.dart file:
import 'package:sentry_flutter/sentry_flutter.dart';
If there are any problems, this line will have a red line underneath it. If all is well, this line will be simply greyed out, just indicating that this import is not yet being used.
I did encounter one hiccup during the process - after adding sentry_flutter as a dependency, running the app on the iOS simulator directly from Android Studio caused errors. Curiously running the app from the terminal was working fine, suggesting possibly some sort of permission issues in Android Studio. This may have just been an issue I'm seeing, but in case anyone else encounters this, I did find a work-around, which was to run Android Studio from the terminal. This can be achieved with the following:
open /Applications/Android\ Studio.app
Once the Sentry dependency is added to your project you should now be able to import it in code:
import 'package:sentry_flutter/sentry_flutter.dart';
You should now be able to use Sentry code in your app. For example, to initialize SentryFlutter, you’ll want to replace main() with an asynchronous version of the method, that passes in the DSN (data source name) for your project:
import 'package:flutter/widgets.dart';
import 'package:sentry_flutter/sentry_flutter.dart';
Future<void> main() async {
await SentryFlutter.init(
(options) {
options.dsn = 'DSN GOES HERE';
options.tracesSampleRate = 1.0; // <- reduce this rate in production
},
appRunner: () => runApp(MyApp()),
);
}
Now you have initialized Sentry, you may choose to create an intentional error to check that Sentry is recording errors correctly. You can find example Dart code for these tasks by looking for the Installation Instructions button in your app's Sentry project online.
Once Sentry has sent event data to the server, you can access information about your app's errors and performance in the web portal. To do this, you'll need to have set up a project for your app in your account on the Sentry website. When creating a project for a native mobile app you would usually have to specify whether you are creating an iOS or Android project. I was curious to know how Sentry would deal with a Flutter project which can work on either platform. Actually, when you are building an app in Flutter, it is not necessary to specify either iOS or Android platforms - instead you can actually specify that you are building a Flutter project, and the same DSN will be relevant for both platforms.
Wrapping up
Well, if I was impressed in the previous article with how smoothly Flutter operates across platforms, with just one codebase requiring both less development time and less maintenance time, experimenting with integrating third party dependencies just confirms for me Flutter's status as a production ready tool for building cross-platform apps. I recommend you give it a try. I for one am looking forward to working with it more.