Logs in Sentry: Now in Open Beta
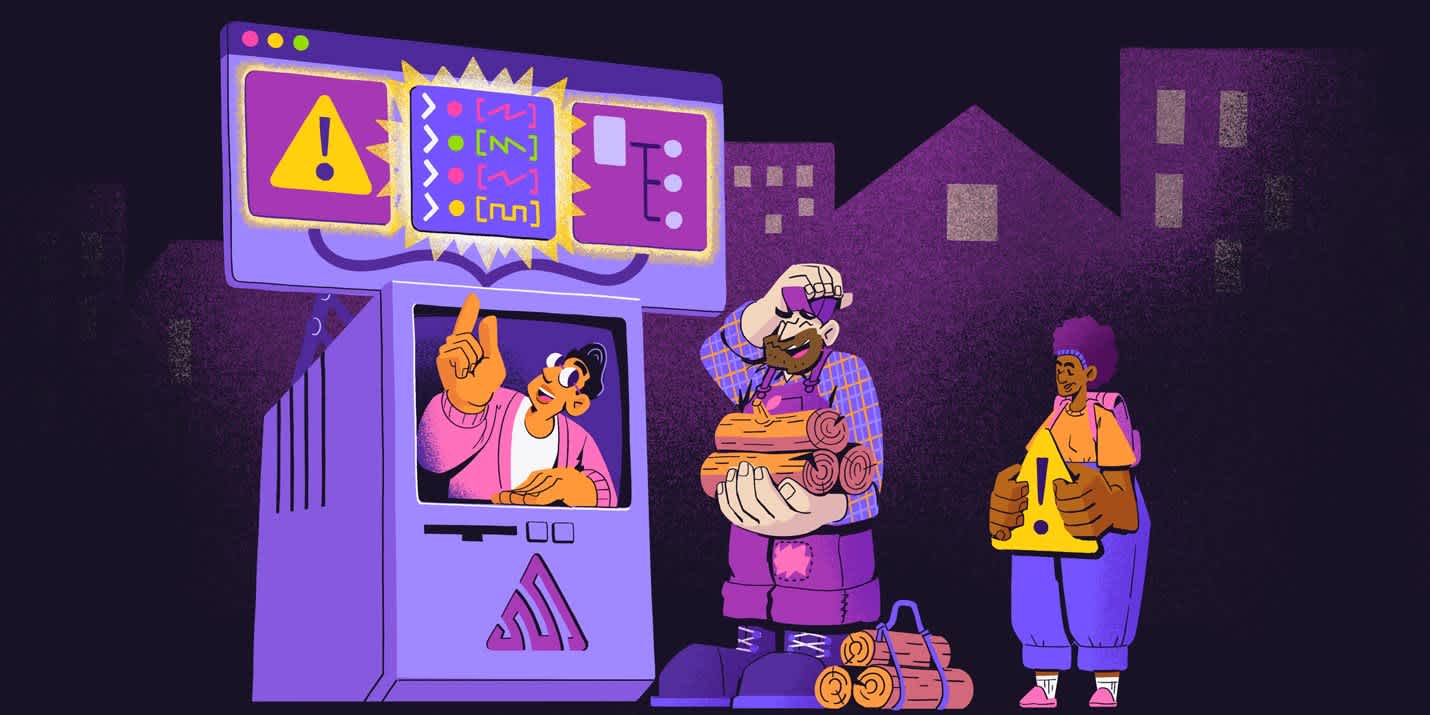
You’re looking at an error in Sentry—a failed payment in your Flask backend or an unexpected null in your Node API. You’ve got the stack trace. The request details. Even the full trace.
What you don’t have: the logs your app emitted right before everything went sideways.
With Sentry Logs (now in open beta), you can send application logs straight to Sentry and see them automatically connected to the errors and traces you already use. That means when something breaks, you don’t just get what happened. You get what led up to it, what happened after, and everything your app had to say about it.
It’s the same debugging workflow you already use—just with more context, and fewer tabs.
Historically, Sentry’s taken a pretty opinionated stance: we’re not a generic log aggregator. There are already a thousand ways to log events from your app. Most of them are pretty good.
What none of them really do is integrate logs directly into your debugging workflow.
Our mission is helping developers find and fix issues faster. Adding logs into the mix wasn’t about chasing another checkbox on a feature matrix—it was about giving you better context at the exact moment you’re dealing with an issue.
Instead of spending hours SSHing into a box, tailing logs, and grepping for "transaction_id"
just to figure out why transactions are failing, you can cut straight to the context that matters. With structured logs in Sentry, you’re not just searching by regexes on logs messages — you’re slicing by real application metadata that matters such as customer_id
, payment_id
, trace_id
. That means you can go from “payments are broken” to “this specific transaction call to Stripe failed because of a timeout” in seconds—without stitching together logs, errors, and traces across different tools
When an error occurs, the logs that preceded it are often the best clues to the root cause.
Sentry automatically links those logs directly to the issue, so when you’re staring at a payment failure error, you’re not starting from scratch. You’ll already have the surrounding logs that show what was passed in, what the upstream service returned, and maybe why your retry logic didn’t kick in.
No need to context switch or manually trace things back from a log aggregator—you’ve got it all right there.
Sometimes things happen that don't trigger an error, like your app suddenly getting slower. While you've always been able to use Sentry's Tracing to see what happened across services and systems, now you can get additional context to see why it happened — with logs right next to the spans they relate to. So the next time you're investigating why your API calls are taking 10x longer than expected, you'll immediately see log messages about retry attempts, fallback paths, or state changes that your code handled without errors but at the cost of performance.
You can start sending logs in under a minute. It works with Python, JavaScript, Go, Ruby, Java and Android — and yes, more are on the way. Below, we’ll show you what this looks like in JavaScript and Python so you can stop flipping between five tools and start seeing logs right next to your errors and traces.
Logs for JavaScript are supported in Sentry JavaScript SDK version 9.17.0
and above. To enable logging, you need to initialize the SDK with the _experiments.enableLogs
option set to true
.
Sentry.init({ dsn: "https://examplePublicKey@o0.ingest.sentry.io/0", // Enable logs to be sent to Sentry _experiments: { enableLogs: true }, });
Once the feature is enabled on the SDK and the SDK is initialized, you can send logs using the Sentry.logger APIs.
const { logger } = Sentry; logger.error(logger.fmt`Uh on, something broke, here's the error: '${error}'`); logger.info(logger.fmt`'${user.username}' added '${product.name}' to cart.`);
If you want to use console or Winston integrations - read our docs to learn more
Logs for Python are supported in Sentry Python SDK version 2.27.0
and above. To enable logging, you need to initialize the SDK with the _experiments.enable_logs
option set to true
.
sentry_sdk.init( dsn="https://examplePublicKey@o0.ingest.sentry.io/0", _experiments={ "enable_logs": True, }, )
Once the feature is enabled on the SDK and the SDK is initialized, you can send logs using the sentry_sdk.logger APIs.
from sentry_sdk import logger as sentry_logger sentry_logger.trace('Starting database connection {database}', database="users") sentry_logger.debug('Cache miss for user {user_id}', user_id=123) sentry_logger.info('Updated global cache') sentry_logger.warning('Rate limit reached for endpoint {endpoint}', endpoint='/api/results/') sentry_logger.error('Failed to process payment. Order: {order_id}. Amount: {amount}', order_id="or_2342", amount=99.99) sentry_logger.fatal('Database {database} connection pool exhausted', database="users")
If you want to integrate with your Python Loggers directly, read our docs to learn more.
We’re just getting started. Here’s what we’re shipping next as we sprint towards general availability:
Log-Based Alerts — get notified on patterns or anomalies
Log Dashboards — visualize key trends and fields
Live Tailing — see new logs as they arrive
More SDKs — including support for PHP, .NET, and several more
Join our GitHub discussion to follow along with updates.
Available on developer, team, and business plans– send logs directly to Sentry and explore them with our new log viewer. No request access. No hidden flags. Just start logging.